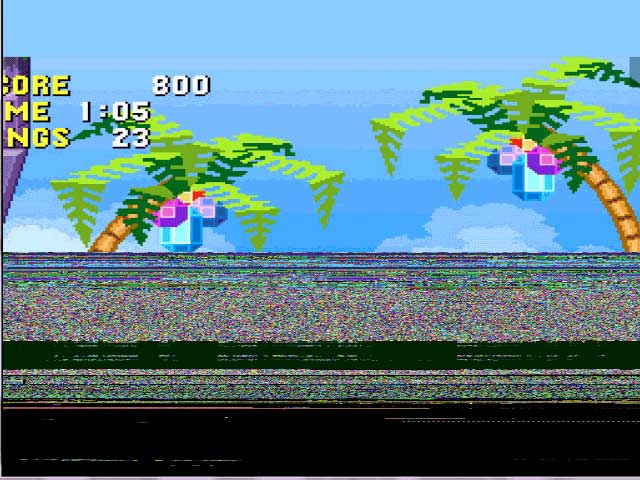
Code: Select all
#include <kos.h>
#include <png/png.h>
/* textures */
pvr_ptr_t back_tex;
pvr_ptr_t front_tex;
/* init background */
void back_init() {
back_tex = pvr_mem_malloc(512 * 512 * 2);
png_to_texture("/rd/back1.png", back_tex, PNG_NO_ALPHA);
front_tex = pvr_mem_malloc(512 * 512 * 2);
pvr_txr_load_ex("/rd/background_tex.tex", front_tex, 512, 512, PVR_TXRLOAD_16BPP);
}
/* draw background png */
void draw_back(void) {
pvr_poly_cxt_t cxt;
pvr_poly_hdr_t hdr;
pvr_vertex_t vert;
pvr_poly_cxt_txr(&cxt, PVR_LIST_OP_POLY, PVR_TXRFMT_RGB565, 512, 512, back_tex, PVR_FILTER_BILINEAR);
pvr_poly_compile(&hdr, &cxt);
pvr_prim(&hdr, sizeof(hdr));
vert.argb = PVR_PACK_COLOR(1.0f, 1.0f, 1.0f, 1.0f);
vert.oargb = 0;
vert.flags = PVR_CMD_VERTEX;
vert.x = 1;
vert.y = 1;
vert.z = 1;
vert.u = 0.0;
vert.v = 0.0;
pvr_prim(&vert, sizeof(vert));
vert.x = 640;
vert.y = 1;
vert.z = 1;
vert.u = 1.0;
vert.v = 0.0;
pvr_prim(&vert, sizeof(vert));
vert.x = 1;
vert.y = 480;
vert.z = 1;
vert.u = 0.0;
vert.v = 1.0;
pvr_prim(&vert, sizeof(vert));
vert.x = 640;
vert.y = 480;
vert.z = 1;
vert.u = 1.0;
vert.v = 1.0;
vert.flags = PVR_CMD_VERTEX_EOL;
pvr_prim(&vert, sizeof(vert));
}
/* draw a texture opaque */
void draw_back2(void) {
pvr_poly_cxt_t cxt;
pvr_poly_hdr_t hdr;
pvr_vertex_t vert;
pvr_poly_cxt_txr(&cxt, PVR_LIST_OP_POLY, PVR_TXRFMT_RGB565, 512, 512, front_tex, PVR_FILTER_BILINEAR);
pvr_poly_compile(&hdr, &cxt);
pvr_prim(&hdr, sizeof(hdr));
vert.argb = PVR_PACK_COLOR(1.0f, 1.0f, 1.0f, 1.0f);
vert.oargb = 0;
vert.flags = PVR_CMD_VERTEX;
vert.x = 1;
vert.y = 256;
vert.z = 10;
vert.u = 0.0;
vert.v = 0.0;
pvr_prim(&vert, sizeof(vert));
vert.x = 640;
vert.y = 256;
vert.z = 10;
vert.u = 1.0;
vert.v = 0.0;
pvr_prim(&vert, sizeof(vert));
vert.x = 1;
vert.y = 480;
vert.z = 10;
vert.u = 0.0;
vert.v = 1.0;
pvr_prim(&vert, sizeof(vert));
vert.x = 640;
vert.y = 480;
vert.z = 10;
vert.u = 1.0;
vert.v = 1.0;
vert.flags = PVR_CMD_VERTEX_EOL;
pvr_prim(&vert, sizeof(vert));
}
/* draw one frame */
void draw_frame(void) {
pvr_wait_ready();
pvr_scene_begin();
pvr_list_begin(PVR_LIST_OP_POLY);
draw_back();
draw_back2();
pvr_list_finish();
pvr_list_begin(PVR_LIST_TR_POLY);
pvr_list_finish();
pvr_scene_finish();
}
/* romdisk */
extern uint8 romdisk_boot[];
KOS_INIT_ROMDISK(romdisk_boot);
int main(void) {
int done = 0;
/* init kos */
pvr_init_defaults();
/* init background */
back_init();
/* keep drawing frames until start is pressed */
while(!done) {
draw_frame();
}
return 0;
}